An Introduction to Solidity Programming for Beginners: The Ultimate Guide to Mastering Smart Contracts
- Krypto Hippo
- Jan 28
- 5 min read
Table of Contents
What is Solidity?
Why Learn Solidity?
Setting Up Your Solidity Development Environment
Basic Solidity Syntax and Structure
Understanding Smart Contracts
Working with Ethereum Blockchain
Deploying Your First Smart Contract
Common Errors in Solidity and How to Fix Them
Best Practices for Writing Solidity Code
Resources for Learning Solidity
Conclusion
FAQs
1. What is Solidity?
Solidity is a powerful programming language used to write smart contracts on the Ethereum blockchain. It is a statically typed, high-level language designed to help developers build decentralized applications (DApps) and deploy them on the blockchain. As blockchain technology continues to grow in popularity, Solidity has become one of the most widely used languages in the cryptocurrency space.
If you’re interested in entering the world of blockchain development or want to learn how to create and deploy smart contracts, Solidity is an essential skill to master.
2. Why Learn Solidity?
With the rise of decentralized finance (DeFi), NFTs, and blockchain-based applications, understanding Solidity is a great way to future-proof your development career. Here's why:
Smart Contracts: Solidity allows you to write code that automatically executes, enforces, and verifies contract terms. These "smart contracts" are essential in creating decentralized applications (DApps).
Growing Market: Ethereum remains the leading blockchain platform for smart contracts. As a result, Solidity developers are in high demand.
Security: Solidity provides developers with the tools necessary to build secure, transparent, and trustless systems. Learning best practices around smart contract security is essential for anyone interested in blockchain technology.
3. Setting Up Your Solidity Development Environment
Before you can start coding in Solidity, you'll need to set up a few tools and environments:
Step 1: Install Node.js
To begin with, you'll need Node.js, which will help you run JavaScript-based tools necessary for Solidity development. You can download it from the official Node.js website.
Step 2: Install Truffle or Hardhat
Truffle and Hardhat are two popular frameworks for Ethereum development. They allow you to easily compile, test, and deploy smart contracts.
Truffle: Truffle is a development framework that simplifies the process of building Ethereum-based applications. It comes with a suite of tools for compiling, testing, and deploying Solidity contracts.
Hardhat: Hardhat is another development environment for building on Ethereum, known for its flexibility and powerful debugging capabilities.
To install either of these frameworks, use the following commands:
For Truffle:
npm install -g truffle
For Hardhat:
npm install --save-dev hardhat
Step 3: Get an Ethereum Wallet
You'll need an Ethereum wallet to interact with the blockchain. Metamask is one of the most popular Ethereum wallets.
4. Basic Solidity Syntax and Structure
Solidity's syntax is similar to JavaScript and C++, making it easier for developers who are familiar with those languages. Here’s a breakdown of the basic syntax:
Contract Structure:
A basic Solidity contract begins with the pragma keyword, which specifies the version of Solidity you're using:
pragma solidity ^0.8.0;
Following this, you'll define the contract using the contract keyword:
contract MyFirstContract {
uint public myNumber;
constructor(uint _myNumber) {
myNumber = _myNumber;
}
function setNumber(uint _myNumber) public {
myNumber = _myNumber;
}
function getNumber() public view returns (uint) {
return myNumber;
}
}
Key Concepts:
State Variables: Variables that are stored on the blockchain (e.g., uint public myNumber).
Constructor: A special function used to initialize state variables when the contract is deployed.
Functions: Functions in Solidity define the behavior of the contract. Functions can be public, private, or internal based on the desired visibility.
5. Understanding Smart Contracts
Smart contracts are self-executing agreements where the terms of the agreement are directly written into lines of code. These contracts run on the blockchain and automatically execute actions when certain conditions are met. Key features include:
Automation: No need for intermediaries.
Security: Transactions are immutable and transparent.
Cost-Effective: Eliminates middlemen, reducing costs and increasing efficiency.
For example, in a simple voting contract, a smart contract could automatically tally votes and announce the winner once the election ends.
6. Working with Ethereum Blockchain
Solidity is designed to work specifically with the Ethereum blockchain, which uses Ethereum Virtual Machine (EVM) to execute smart contracts. Understanding the Ethereum blockchain is crucial for developing smart contracts.
Transactions and Gas:
Ethereum transactions require "gas," which is a unit of computation cost to perform actions like storing data or transferring funds. Developers must optimize their code to use as little gas as possible to save on transaction costs.
7. Deploying Your First Smart Contract
After writing your smart contract, the next step is to deploy it to the Ethereum blockchain. You can do this on a testnet (e.g., Rinkeby) before deploying to the main Ethereum network.
Deploying Using Remix IDE:
Remix is an online Solidity IDE that allows you to write, test, and deploy Solidity contracts in your browser.
Go to the Remix IDE website, create your contract, and use the "Deploy & Run Transactions" tab to deploy your contract.
Deploying Using Truffle or Hardhat:
If you prefer to work locally or build more complex DApps, you can deploy your contract using Truffle or Hardhat with an Ethereum wallet like Metamask to sign transactions.
8. Common Errors in Solidity and How to Fix Them
Solidity programming, like any development process, can come with its share of errors. Here are some common issues and solutions:
Out-of-Gas Error: This occurs when a function runs out of gas due to inefficient code or a state-changing operation.
Fix: Optimize your code and make sure transactions don’t use too much gas.
Reentrancy Attack: This happens when an external contract makes a recursive call back to your contract.
Fix: Use the "checks-effects-interactions" pattern to prevent reentrancy.
Unchecked Call Return Value: When interacting with other contracts, always check the return value of external function calls to ensure the call was successful.
9. Best Practices for Writing Solidity Code
To write secure and efficient Solidity code, adhere to the following best practices:
Use Safe Math: To prevent overflow and underflow errors, always use SafeMath library functions.
Keep Functions Short: Shorter functions are easier to test, debug, and understand.
Limit Gas Usage: Optimize your contract code to minimize gas costs for users.
10. Resources for Learning Solidity
There are a wealth of resources available to help you learn Solidity:
Official Solidity Documentation: Solidity Docs
CryptoZombies: An interactive tutorial to learn Solidity by creating a zombie-themed game (CryptoZombies).
Solidity by Example: A collection of examples and explanations for Solidity programming (Solidity by Example).
11. Conclusion
An Introduction to Solidity Programming for Beginners: The Ultimate Guide to Mastering Smart Contracts. Learning Solidity is a valuable skill for anyone interested in blockchain development.
With Solidity, you can build decentralized applications, automate transactions, and take part in the growing world of DeFi. By setting up a development environment, understanding the syntax, and practicing on testnets, you can begin your journey into smart contract development.
FAQs An Introduction to Solidity Programming for Beginners: The Ultimate Guide to Mastering Smart Contracts
Q: How long does it take to learn Solidity?
A: The time it takes to learn Solidity depends on your background in programming. If you’re already familiar with JavaScript or other programming languages, you can start writing simple contracts in a few weeks. For complete beginners, it may take a few months to become proficient.
Q: Do I need to know blockchain technology to learn Solidity?
A: While knowledge of blockchain concepts like Ethereum and smart contracts is helpful, it’s not required to start learning Solidity. You can begin with Solidity and gradually familiarize yourself with the underlying blockchain technology.
Q: Can I test my Solidity contracts without using real ETH?
A: Yes! You can use Ethereum testnets like Rinkeby or Kovan to test your contracts without using real cryptocurrency.
Q: Is Solidity the only language for writing smart contracts?
A: No. While Solidity is the most widely used language for Ethereum smart contracts, other blockchains like EOS and TRON use different languages. For example, EOS uses C++.
By following this guide, you should have a solid foundation in Solidity programming. Happy coding!
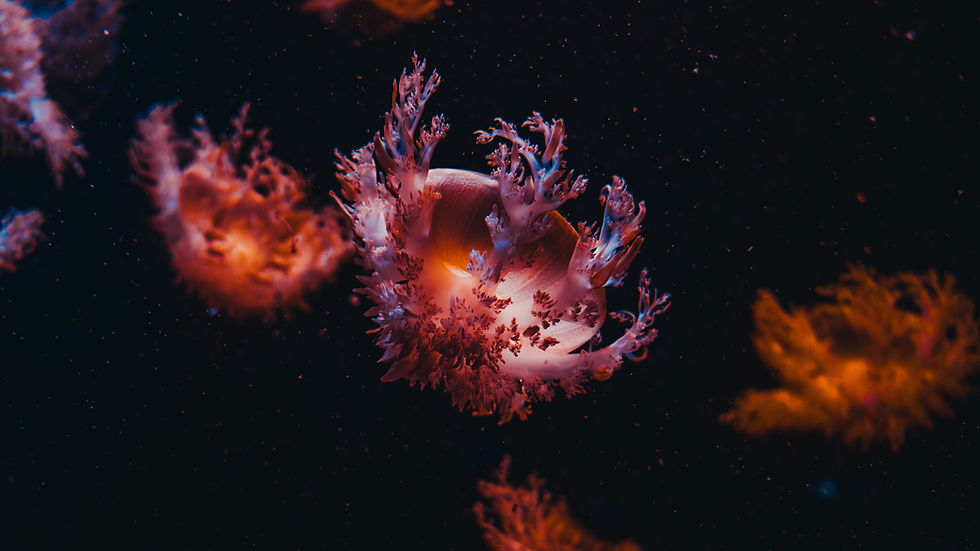