Building a Full-Stack Blockchain Application: A Developer’s Guide
- Krypto Hippo
- Feb 25
- 7 min read
Table of Contents
Introduction: The Rise of Blockchain Applications
What is a Full-Stack Blockchain Application?
Key Components of a Full-Stack Blockchain Application
3.1 Frontend Development
3.2 Backend Development
3.3 Smart Contracts
Tools and Technologies for Building a Blockchain Application
4.1 Ethereum and Smart Contracts
4.2 Development Frameworks: Truffle and Hardhat
4.3 Frontend Technologies: React.js and Web3.js
4.4 Backend Frameworks: Node.js and Express
Setting Up the Development Environment
5.1 Installing Node.js and NPM
5.2 Installing Ethereum and Smart Contract Tools
Developing the Smart Contract
6.1 Writing Your First Smart Contract in Solidity
6.2 Testing and Deploying Smart Contracts
Developing the Frontend
7.1 Connecting the Frontend with Web3.js
7.2 Interacting with Smart Contracts from the Frontend
Developing the Backend
8.1 Backend Integration with the Blockchain
8.2 Setting Up a Backend API for Blockchain Interaction
Deploying Your Blockchain Application
9.1 Deploying Smart Contracts to a Testnet
9.2 Deploying the Full Application to Production
Best Practices for Full-Stack Blockchain Development
Conclusion: The Future of Full-Stack Blockchain Development
Frequently Asked Questions (FAQs)
1. Introduction: The Rise of Blockchain Applications
Blockchain technology has evolved far beyond its origins with Bitcoin and cryptocurrency. Today, blockchain serves as the backbone for decentralized applications (dApps), smart contracts, and various blockchain-powered ecosystems. As the demand for decentralized solutions continues to rise, developers are finding new opportunities in building blockchain-based applications.
A full-stack blockchain application is a software solution that leverages blockchain technology for its backend, integrates smart contracts, and includes a user-friendly interface for interaction. These applications span the entire development stack, from the front-end user interface to the back-end services interacting with the blockchain.
In this guide, we’ll walk you through the essential steps for building a full-stack blockchain application, including key technologies, tools, and best practices. Whether you're a seasoned developer or new to blockchain, this guide will help you understand how to build, deploy, and manage a blockchain-based application.
2. What is a Full-Stack Blockchain Application?
A full-stack blockchain application consists of several interconnected layers of technology that combine blockchain, smart contracts, and front-end and back-end systems into a cohesive application. It includes:
Frontend Development: The user-facing interface where users interact with the application.
Backend Development: The server-side logic, APIs, and databases (if applicable) used to manage application data and blockchain interaction.
Smart Contracts: Self-executing contracts with predefined rules and logic, deployed on a blockchain network like Ethereum.
This structure mirrors traditional web applications, but with blockchain at the core, providing enhanced security, decentralization, and transparency.
3. Key Components of a Full-Stack Blockchain Application
3.1 Frontend Development
The frontend of a full-stack blockchain application is responsible for delivering the user interface (UI) and user experience (UX). Users interact with the dApp using a web browser, so frontend development typically involves HTML, CSS, JavaScript, and frameworks like React.js.
To interact with blockchain functionality, the frontend will need to integrate with Web3.js or Ethers.js libraries, which enable communication between the frontend and blockchain networks.
3.2 Backend Development
The backend of a full-stack blockchain app is responsible for handling business logic, managing databases, and interfacing with blockchain nodes or APIs. In the context of blockchain, the backend communicates with blockchain nodes to query data or interact with smart contracts.
Popular backend technologies for blockchain applications include Node.js, Express.js, and Python, often combined with databases (SQL or NoSQL) to store non-blockchain data. In decentralized apps, the backend may be simplified or even absent, as blockchain smart contracts themselves serve as business logic.
3.3 Smart Contracts
Smart contracts are the heart of blockchain-based applications. These are self-executing agreements written in code that are deployed on a blockchain platform like Ethereum. Smart contracts automate business logic without the need for intermediaries.
For example, in a decentralized finance (DeFi) application, smart contracts control the flow of assets and define rules for lending, borrowing, and trading.
4. Tools and Technologies for Building a Blockchain Application
4.1 Ethereum and Smart Contracts
Ethereum is one of the most popular blockchain platforms for building decentralized applications. It provides the tools and infrastructure to write and deploy smart contracts in a language called Solidity.
Ethereum’s Ethereum Virtual Machine (EVM) runs smart contracts, and Web3.js or Ethers.js libraries allow your frontend to interact with Ethereum’s blockchain.
4.2 Development Frameworks: Truffle and Hardhat
Two widely used frameworks for developing Ethereum smart contracts are Truffle and Hardhat.
Truffle: A comprehensive development framework that includes tools for compiling, testing, and deploying smart contracts.
Hardhat: A newer development environment that provides flexibility for building, testing, and deploying Ethereum smart contracts with a focus on ease of use and debugging.
4.3 Frontend Technologies: React.js and Web3.js
For the frontend, React.js is a popular choice due to its flexibility, fast rendering, and scalability. It allows developers to build responsive UIs that integrate seamlessly with blockchain-based backends.
To connect the frontend with the blockchain, developers use Web3.js or Ethers.js, libraries that provide a way for JavaScript applications to interact with the blockchain. These libraries enable the frontend to read from and write to the blockchain, as well as sign transactions.
4.4 Backend Frameworks: Node.js and Express
For the backend, Node.js is a popular choice due to its non-blocking, event-driven architecture, which makes it suitable for handling asynchronous operations that are common in blockchain interactions.
Express.js is often used alongside Node.js to build RESTful APIs, which can be used for interacting with the blockchain, handling data from users, or storing off-chain data (e.g., user profiles).
5. Setting Up the Development Environment
5.1 Installing Node.js and NPM
Before diving into blockchain development, you’ll need to set up your development environment. Start by installing Node.js and npm (Node Package Manager), which are essential for building and managing blockchain applications.
Go to the official Node.js website and download the latest stable version.
Install Node.js, which will automatically install npm.
Verify installation by running the following commands in your terminal: node -v npm -v
5.2 Installing Ethereum and Smart Contract Tools
To interact with the Ethereum network and write smart contracts, install the following tools:
Truffle: Install Truffle globally with npm: npm install -g truffle
Ganache: Ganache is a local blockchain for testing and deploying Ethereum smart contracts. You can download it from the Truffle website or use Ganache CLI.
Hardhat: For a more customizable environment, install Hardhat:
npm install --save-dev hardhat
6. Developing the Smart Contract
6.1 Writing Your First Smart Contract in Solidity
Solidity is the most widely used language for writing smart contracts on the Ethereum network. A basic smart contract might look like this:
pragma solidity ^0.8.0;
contract SimpleStorage {
uint256 storedData;
function set(uint256 x) public {
storedData = x;
}
function get() public view returns (uint256) {
return storedData;
}
}
This contract allows users to set and get a value. Once written, you can compile and deploy it to the Ethereum testnet or mainnet.
6.2 Testing and Deploying Smart Contracts
Using frameworks like Truffle or Hardhat, you can test your smart contract in a local blockchain environment (e.g., Ganache) or on a testnet (e.g., Rinkeby or Ropsten). The process includes compiling the smart contract, writing tests, and deploying it to the network.
7. Developing the Frontend
7.1 Connecting the Frontend with Web3.js
The frontend interacts with the blockchain through Web3.js or Ethers.js. These libraries enable the frontend to send transactions, query blockchain data, and interact with smart contracts. Example:
const Web3 = require('web3');
const web3 = new Web3(window.ethereum);
await window.ethereum.request({ method: 'eth_requestAccounts' });
This code connects the frontend to the user's Ethereum wallet (e.g., MetaMask) and prompts them to sign transactions.
7.2 Interacting with Smart Contracts from the Frontend
To interact with a smart contract, you can use Web3.js to call the contract's functions. For example:
const contract = new web3.eth.Contract(abi, contractAddress);
const value = await contract.methods.get().call();
console.log(value);
This retrieves data from the blockchain and displays it in the UI.
8. Developing the Backend
8.1 Backend Integration with the Blockchain
The backend may be used to interact with the blockchain to fetch data or submit transactions. Node.js can help manage asynchronous calls to the blockchain via Web3.js.
8.2 Setting Up a Backend API for Blockchain Interaction
You can create a RESTful API using Express.js to interact with the blockchain. For example, an endpoint to retrieve data from a smart contract might look like:
app.get('/get-value', async (req, res) => {
const value = await contract.methods.get().call();
res.send({ value });
});
9. Deploying Your Blockchain Application
9.1 Deploying Smart Contracts to a Testnet
Once your smart contract is written and tested, deploy it to an Ethereum testnet (e.g., Rinkeby). Use Truffle or Hardhat for deployment:
truffle migrate --network rinkeby
9.2 Deploying the Full Application to Production
After thorough testing, deploy your frontend to a hosting platform like Netlify or Vercel and the backend to Heroku or AWS. Ensure that your smart contract is deployed to the Ethereum mainnet before going live.
10. Best Practices for Full-Stack Blockchain Development
Use Version Control: Always use Git for version control to track changes and collaborate effectively.
Write Unit Tests: Test smart contracts rigorously using testing frameworks like Mocha or Chai.
Optimize Gas Usage: Be mindful of gas costs when writing smart contracts to ensure efficiency.
Security Audits: Conduct security audits of your smart contracts to prevent vulnerabilities.
Stay Updated: The blockchain space is evolving rapidly, so stay up-to-date with the latest trends and tools.
11. Conclusion: The Future of Full-Stack Blockchain Development
Building a Full-Stack Blockchain Application: A Developer’s Guide. Building a full-stack blockchain application is a complex but rewarding process. It involves mastering blockchain development tools, smart contracts, and frontend technologies. As the blockchain ecosystem continues to grow, the demand for decentralized applications (dApps) will only increase.
By following the steps outlined in this guide, developers can successfully create and deploy full-stack blockchain applications that solve real-world problems and drive innovation in the blockchain space.
Frequently Asked Questions (FAQs) Building a Full-Stack Blockchain Application: A Developer’s Guide
1. What programming languages do I need to learn for blockchain development?
The primary language for smart contracts on Ethereum is Solidity. For the frontend, you'll need to know JavaScript, and for the backend, Node.js and Express.js are commonly used.
2. How do I deploy a smart contract to Ethereum?
You can deploy smart contracts using tools like Truffle or Hardhat. These frameworks provide commands to deploy to both testnets and the Ethereum mainnet.
3. What is Web3.js?
Web3.js is a JavaScript library that allows web applications to interact with Ethereum blockchain networks. It is used to send transactions and interact with smart contracts from the frontend.
4. What is the best blockchain for dApp development?
While Ethereum is the most popular blockchain for dApps, other blockchains like Binance Smart Chain (BSC), Solana, and Polygon also support decentralized applications.
5. How can I ensure the security of my smart contract?
Conduct regular security audits, use unit testing frameworks, and follow best practices for coding and gas optimization to ensure smart contract security.
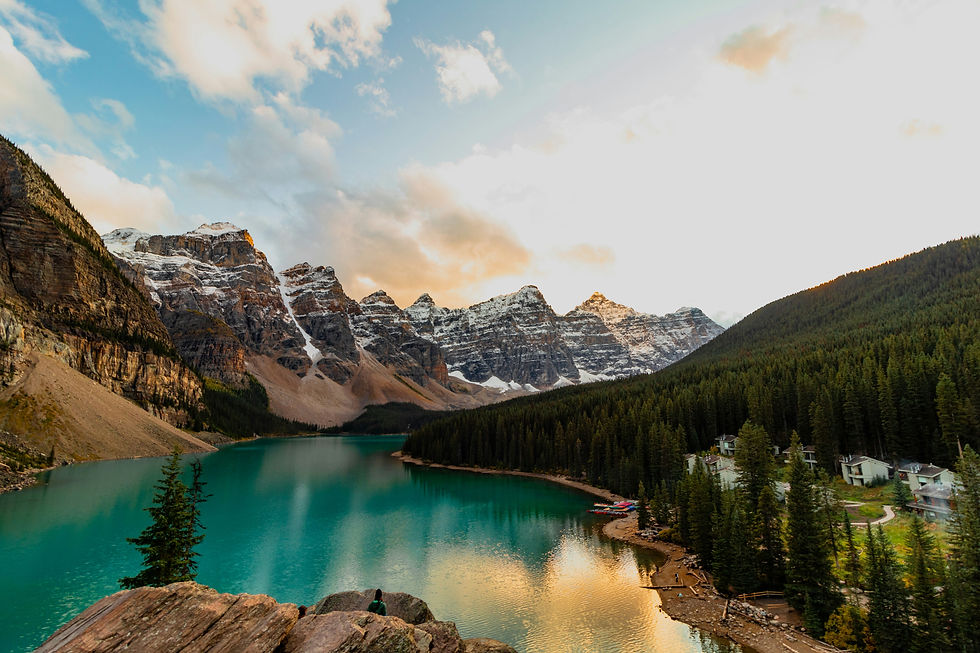